Published
- 2 min read
How To Parse A JSON With SwiftyJSON
by Hong Cheung
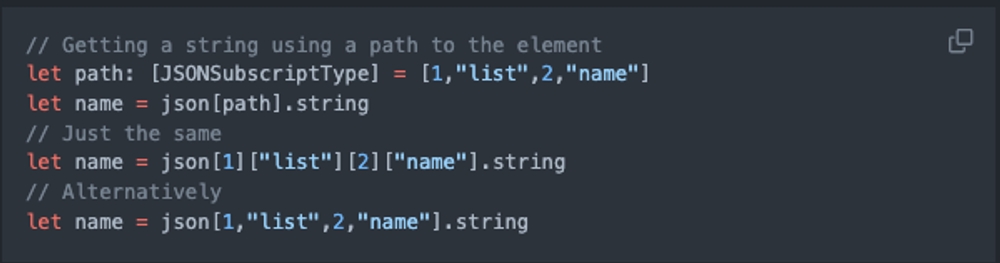
What is SwiftyJSON?
SwiftyJSON is a third party library for decoding JSON file more easily in your Xcode project.
Why do you need it?
It is considered the best practise to encode and decode JSON with predefined type. However, the downside of using the built-in JSONDecoder is that it is a hassle to deal with dynamic JSON. Even if you could manage to get it done, it would still need a lot of work just to get it right, which is not ideal.
Dynamic JSON example
Image you have a JSON looks like this:
{
"data": {
"variable_1": "some text"
}
}
Where the variable_1 key will be changed dynamically, you cannot just decode the data simply using data.title. What if it’s data.description next time?
In JavaScript, we can easily do this:
let dynamicKey = 'variable'
const json = JSON.parse(JSONObejct)
console.log(json.data[`${dynamicKey}_1`])
You can access the value just by putting a dynamic string in to [] behind data. However, you cannot do this in Swift.
That’s why SwiftyJSON comes into play.
To Parse a JSON using SwiftyJSON
With the help of SwiftyJSON, you can do this:
let json = JSON(data: JSONObejct)
let dynamicVar = "variable_1"
if let extracted = json[0]["data"]["\(dynamicVar)"].string { // It's wrapped with Optional automatically
//Now you got your value inside data.variable_1, just change your variable value for dynamicVar as needed.
print(extracted) // some text
}
It is pretty much the same way you do in JavaScript.
Direct and simple.
Bottom line
Even though the library still works, the library is not maintained anymore. The latest commit was 2 years ago.
I will still be using it for my projects though, because it saves me a lot of time and hassle.
Almost a must-have library for dealing with JSON.